In sum;
In function.php of your theme:
function my_theme_join_mytable_to_WPQuery($join) { global $wpdb; $join .= " LEFT JOIN {$wpdb->prefix}mytable ON $wpdb->posts.ID = {$wpdb->prefix}mytable.postid "; return $join; } add_filter('posts_join', 'my_theme_join_mytable_to_WPQuery');
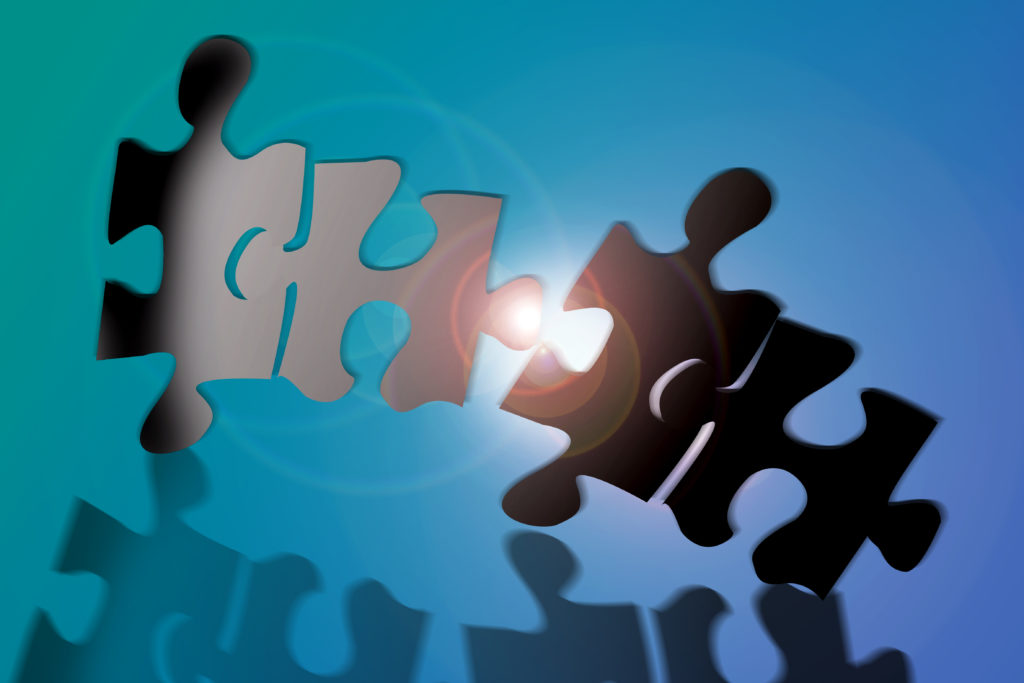
Jigsaw puzzle symbolizing joined tables © Ajv123ajv | Dreamstime Stock Photos
Explanation:
If you want to join tables in wordpress whether left join or inner to wordpress posts query. Say if you need to display some custom rating, game scores or any other data you want to associate with the table. It’s more efficient to join the table to the posts database table using wordpress function hooks. Rather than fetching your custom tables inside the template on the fly.
The benefit of using wordpress hooks and functions is not just efficiency. in using database queries. but also you can let other plugins you install like database caches or other processes to work with your custom tables without any extra steps.
You can use functions.php in your theme or your custom plugin to hook to the posts_join wordpress api. you need to register table join hook filter with the following command:
add_filter(‘posts_join’, ‘my_theme_join_mytable_to_WPQuery’);
What this does, it tells wordpress to call your custom function my_theme_join_mytable_to_WPQuery when building the posts fetching query. so every time a user views your site and sees the list of posts or search posts. the function will be called before querying the database. The filter hook passes the join statement sent by user.
Then add a function with the custom name you’ve chosen earlier: my_theme_join_mytable_to_WPQuery
function my_theme_join_mytable_to_WPQuery($join) { }
The join variable is a string that contains the join statements added by other filters or wordpress. you need concatenate to it. as:
$join .= " LEFT JOIN MYTABLE ON MYTABLE.id = wp_posts.post.id = MYTABLE.postid";
then you must return join back
return $join;
It’s better however to use wordpress table names and prefixes. it’s possible the prefix of tables not wp_ or table names for wordpress change. instead $wpdb->prefix to get the prefix and $wpdb->posts to get wordpress post table (this includes the prefix). I assume your custom table also has the same wordpress prefix as it should.
therefore your table becomes {$wpdb->prefix}mytable
You need to import the variable $wpdb from the global scope. using global $wpdb.
Finally it would look like this:
function my_theme_join_mytable_to_WPQuery($join) { global $wpdb; $join .= " LEFT JOIN {$wpdb->prefix}mytable ON $wpdb->posts.ID = {$wpdb->prefix}mytable.postid "; return $join; } add_filter('posts_join', 'my_theme_join_mytable_to_WPQuery');